When it comes to web development, having a robust and flexible framework can make all the difference. Flask software, a lightweight microframework for Python, is one such tool that developers love due to its simplicity, flexibility, and ease of use. In this guide, we will explore what Flask software is, why it’s so popular among developers, and how you can leverage its features to build scalable web applications.
What is Flask Software?
Flask is a Python-based web framework that provides the necessary tools and libraries for building web applications. Unlike full-stack frameworks like Django, Flask software is categorized as a microframework because it doesn’t include many built-in features or components like database systems or form validation. This minimalism allows developers to pick and choose libraries and tools as needed, making Flask extremely versatile for both small and large projects.
Despite its minimalistic approach, Flask software remains powerful and scalable. It’s designed with simplicity in mind but doesn’t compromise on performance. You can use Flask to develop anything from single-page applications (SPAs) to more complex, API-driven web services.
Why Use Flask Software?
1. Simplicity and Minimalism
One of the key reasons developers choose Flask is because of its simplicity. Flask allows you to build a web application with just a few lines of Python code. There’s no need for complicated setups, and the framework doesn’t enforce any project layout or structure. This gives developers the flexibility to structure their applications the way they want.
2. Flexibility and Extensibility
Unlike larger frameworks, Flask software doesn’t come with built-in components such as an ORM (Object-Relational Mapper) or form handling. Instead, it gives developers the freedom to choose whichever libraries or tools they prefer. You can easily integrate Flask with external libraries and tools to extend its functionality as your project grows.
3. Easy to Learn for Beginners
Flask software is an excellent starting point for developers who are new to web development. Since it only provides the basics and doesn’t overload the developer with too many options out of the box, it’s easy to grasp. Beginners can focus on learning Python while building real-world web applications without getting overwhelmed by the intricacies of a full-stack framework.
4. Lightweight and Fast
Flask is designed to be lightweight, meaning that it doesn’t require many resources to run. This makes Flask ideal for projects where speed and performance are critical. Because Flask doesn’t include unnecessary components, the overall framework is more efficient, which contributes to faster load times and better performance.
5. Strong Community Support
Despite its minimalist design, Flask software has a strong and active community. This means that if you ever run into problems, there’s a wealth of tutorials, guides, and forums available to help you out. Flask’s documentation is also highly praised for being clear and comprehensive, which is a big plus for both beginners and experienced developers.
Key Features of Flask Software
Although Flask software is a minimalistic framework, it still offers several powerful features that make it an excellent choice for web development:
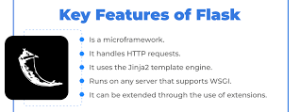
1. Routing System
Flask’s routing system allows developers to map specific URLs to Python functions. This is one of the core features of any web framework, and Flask makes it easy to implement. You can define routes using decorators, making the process both intuitive and efficient.
from flask import Flask
app = Flask(__name__)
def home():
return “Welcome to Flask Software!”
if __name__ == ‘__main__’:
app.run(debug=True)
2. Jinja2 Templating
Flask software uses the Jinja2 template engine, which allows you to dynamically generate HTML pages based on the data passed from your Python code. Jinja2 makes it easy to separate your Python logic from your HTML structure, promoting clean and maintainable code.
<html>
<head>
<title>Flask Example</title>
</head>
<body>
<h1>{{ title }}</h1>
<p>{{ message }}</p>
</body>
</html>
3. Support for APIs
Flask software is widely used for creating RESTful APIs. Its lightweight nature and flexibility make it perfect for developing API-driven applications. With just a few lines of code, you can create endpoints to serve data in formats such as JSON.
from flask import Flask, jsonify
app = Flask(__name__)
def api_example():
data = {‘message’: ‘Hello, Flask software!’}
return jsonify(data)
4. Extension Support
One of the standout features of Flask software is its support for extensions. Extensions allow you to add features such as database integration, authentication, form handling, and more to your Flask application. Some popular Flask extensions include:
- Flask-SQLAlchemy: Adds ORM support for relational databases.
- Flask-WTF: Simplifies form validation and handling.
- Flask-Login: Provides user authentication mechanisms.
- Flask-Mail: Enables email sending capabilities.
These extensions can be easily integrated into your Flask application, allowing you to enhance its functionality without compromising its lightweight nature.
5. Secure Cookies and Session Management
Flask software provides built-in support for secure cookies, which are essential for maintaining user sessions in web applications. You can use Flask’s session management to store and retrieve user data securely.
from flask import Flask, session
app = Flask(__name__)
app.secret_key = ‘your_secret_key’
def set_session():
session[‘username’] = ‘FlaskUser’
return ‘Session data set’
def get_session():
username = session.get(‘username’)
return f’Logged in as {username}‘
Flask Software Use Cases
Flask software is ideal for a wide range of applications due to its versatility. Here are some common use cases where Flask excels:
1. Prototyping
Because of its lightweight design, Flask software is excellent for building prototypes and minimum viable products (MVPs). Developers can quickly spin up an application to test ideas, gather feedback, and iterate without dealing with the overhead of a full-stack framework.
2. Small to Medium-Scale Web Applications
For small and medium-scale web applications, Flask software provides just the right balance of flexibility and simplicity. It allows developers to build scalable applications without imposing too many constraints.
3. RESTful APIs
Flask software is one of the go-to frameworks for developing RESTful APIs. Its minimal design makes it easy to create fast, efficient APIs that can serve data to web and mobile applications.
4. Microservices
Flask software is a popular choice for building microservices due to its lightweight nature and modular design. Flask’s flexibility allows developers to build independent services that can communicate with each other, providing a scalable solution for complex systems.
Getting Started with Flask Software
Getting started with Flask software is simple. Here’s a basic setup guide to help you get up and running:
Step 1: Install Flask
To install Flask, you’ll need to have Python installed on your system. Once you have Python set up, you can install Flask using pip:
pip install Flask
Step 2: Create a Basic Flask App
After installing Flask software, you can create a simple application:
from flask import Flask
app = Flask(__name__)
def home():
return “Hello, Flask software!”
if __name__ == ‘__main__’:
app.run(debug=True)
Save this code in a file (e.g., app.py
), and run it using the following command:
python app.py
You can now access your Flask application by navigating to http://127.0.0.1:5000/
in your browser.
Conclusion
Flask software is a powerful and flexible framework that caters to developers who need simplicity and control. Its lightweight design, combined with extensive support for extensions, makes it an ideal choice for a wide range of web development projects. Whether you’re building a small-scale web app, a RESTful API, or a microservice, Flask software has the tools you need to succeed.
By allowing developers to focus on writing clean, efficient code without the bloat of unnecessary features, Flask software continues to be a popular choice in the Python ecosystem. Its simplicity, flexibility, and community support make it an excellent framework for both beginners and seasoned developers alike.